C# HashSet - c# - c# tutorial - c# net
What in C# Hashset ?
- C# HashSet class can be used to store, remove or view elements.
- It does not store duplicate elements.
- It is suggested to use HashSet class if you have to store only unique elements.
- It is found in System.Collections.Generic namespace.
- This is an optimized set collection. It helps eliminates duplicate strings or elements in an array. It is a set that hashes its contents.
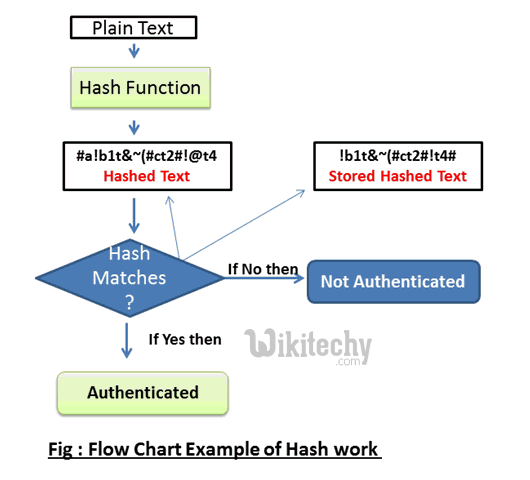
Csharp hash function
Syntax:
[SerializableAttribute]
[HostProtectionAttribute(SecurityAction.LinkDemand, MayLeakOnAbort = true)]
public class HashSet<T> : ICollection<T>, IEnumerable<T>, IEnumerable,
ISerializable, IDeserializationCallback, ISet<T>, IReadOnlyCollection<T>
C# HashSet<T> example
- Let's see an example of generic HashSet<T> class that stores elements using Add() method and iterates elements using for-each loop.
using System;
using System.Collections.Generic;
public class HashSetExample
{
public static void Main(string[] args)
{
// Create a set of strings
var names = new HashSet<string>();
names.Add("Sonoo");
names.Add("Ankit");
names.Add("Peter");
names.Add("Irfan");
names.Add("Ankit");//will not be added
// Iterate HashSet elements using foreach loop
foreach (var name in names)
{
Console.WriteLine(name);
}
}
}
C# examples - Output :
Sonoo
Ankit
Peter
Irfan
Read Also
dot net developer internship , best sql training institutes in chennai , dot net internshipC# HashSet<T> example 2:
- Let's see another example of generic HashSet<T> class that stores elements using Collection initializer.
using System;
using System.Collections.Generic;
public class HashSetExample
{
public static void Main(string[] args)
{
// Create a set of strings
var names = new HashSet<string>(){"Sonoo", "Ankit", "Peter", "Irfan"};
// Iterate HashSet elements using foreach loop
foreach (var name in names)
{
Console.WriteLine(name);
}
}
}
C# examples - Output :
Sonoo
Ankit
Peter
Irfan
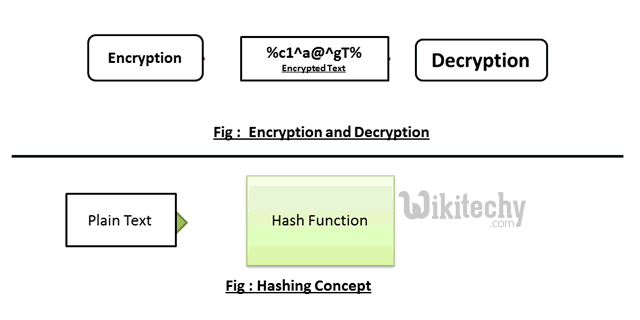
Csharp encryption hash function