C# Constructor - c# - c# tutorial - c# net
What are the types of constructor in C# ?
- In C#, constructor is a special method which is invoked automatically at the time of object creation.
- It is used to initialize the data members of new object generally.
- The constructor in C# has the same name as class or struct.
- Whenever a class or struct is created, its constructor is called.
- A class or struct may have multiple constructors that take different arguments.
- Constructors enable the programmer to set default values, limit instantiation, and write code that is flexible and easy to read
- There can be two types of constructors in C#.
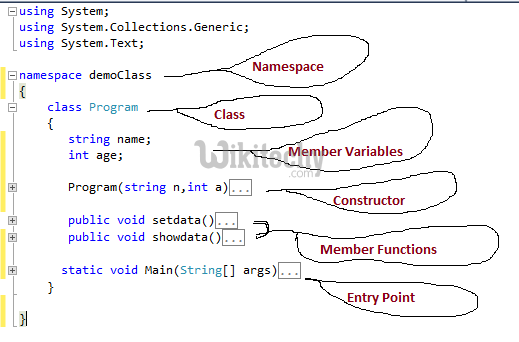
class-object-contructor-member-function-in-csharp in c# Example
- Default constructor
- Parameterized constructor
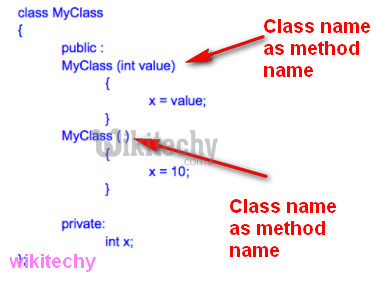
Constructor
Default Constructor
- A constructor which has no argument is known as default constructor. It is invoked at the time of creating object.
C# Default Constructor Example: Having Main() within class
using System;
public class Employee
{
public Employee()
{
Console.WriteLine("Default Constructor Invoked");
}
public static void Main(string[] args)
{
Employee e1 = new Employee();
Employee e2 = new Employee();
}
}
C# examples - Output :
Default Constructor Invoked
Default Constructor Invoked
C# Default Constructor Example: Having Main() in another class
- Let's see another example of default constructor where we are having Main() method in another class.
using System;
public class Employee
{
public Employee()
{
Console.WriteLine("Default Constructor Invoked");
}
}
class TestEmployee{
public static void Main(string[] args)
{
Employee e1 = new Employee();
Employee e2 = new Employee();
}
}
C# examples - Output :
Default Constructor Invoked
Default Constructor Invoked
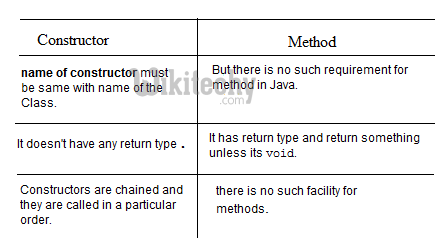
Constructor vs method csharp in c# Example
Parameterized Constructor
- A constructor which has parameters is called parameterized constructor. It is used to provide different values to distinct objects.
using System;
public class Employee
{
public int id;
public String name;
public float salary;
public Employee(int i, String n,float s)
{
id = i;
name = n;
salary = s;
}
public void display()
{
Console.WriteLine(id + " " + name+" "+salary);
}
}
class TestEmployee{
public static void Main(string[] args)
{
Employee e1 = new Employee(101, "Sonoo", 890000f);
Employee e2 = new Employee(102, "Mahesh", 490000f);
e1.display();
e2.display();
}
}
C# examples - Output :
101 Sonoo 890000
102 Mahesh 490000