C# Sealed - c# - c# tutorial - c# net
What is sealed keyword in C# ?
- C# sealed keyword applies restrictions on the class and method.
- If you create a sealed class, it cannot be derived.
- If you create a sealed method, it cannot be overridden.
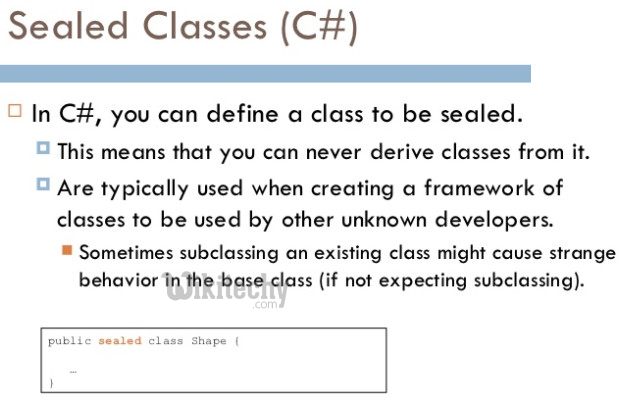
Sealed class csharp in c# Example
Note: Structs are implicitly sealed therefore they can't be inherited.
C# Sealed class
- C# sealed class cannot be derived by any class. Let's see an example of sealed class in C#.
using System;
sealed public class Animal{
public void eat() { Console.WriteLine("eating..."); }
}
public class Dog: Animal
{
public void bark() { Console.WriteLine("barking..."); }
}
public class TestSealed
{
public static void Main()
{
Dog d = new Dog();
d.eat();
d.bark();
}
}
C# examples - Output :
Compile Time Error: 'Dog': cannot derive from sealed type 'Animal'
C# Sealed method
- The sealed method in C# cannot be overridden further. It must be used with override keyword in method.
- Let's see an example of sealed method in C#.
using System;
public class Animal{
public virtual void eat() { Console.WriteLine("eating..."); }
public virtual void run() { Console.WriteLine("running...");}
}
public class Dog: Animal
{
public override void eat() { Console.WriteLine("eating bread..."); }
public sealed override void run() {
Console.WriteLine("running very fast...");
}
}
public class BabyDog : Dog
{
public override void eat() { Console.WriteLine("eating biscuits..."); }
public override void run() { Console.WriteLine("running slowly..."); }
}
public class TestSealed
{
public static void Main()
{
BabyDog d = new BabyDog();
d.eat();
d.run();
}
}
C# examples - Output :
Compile Time Error: 'BabyDog.run()': cannot override inherited member 'Dog.run()' because it is sealed
Note: Local variables can't be sealed.
using System;
public class TestSealed
{
public static void Main()
{
sealed int x = 10;
x++;
Console.WriteLine(x);
}
}
C# examples - Output :
Compile Time Error: Invalid expression term 'sealed'