C# foreach loops - c# - c# tutorial - c# net
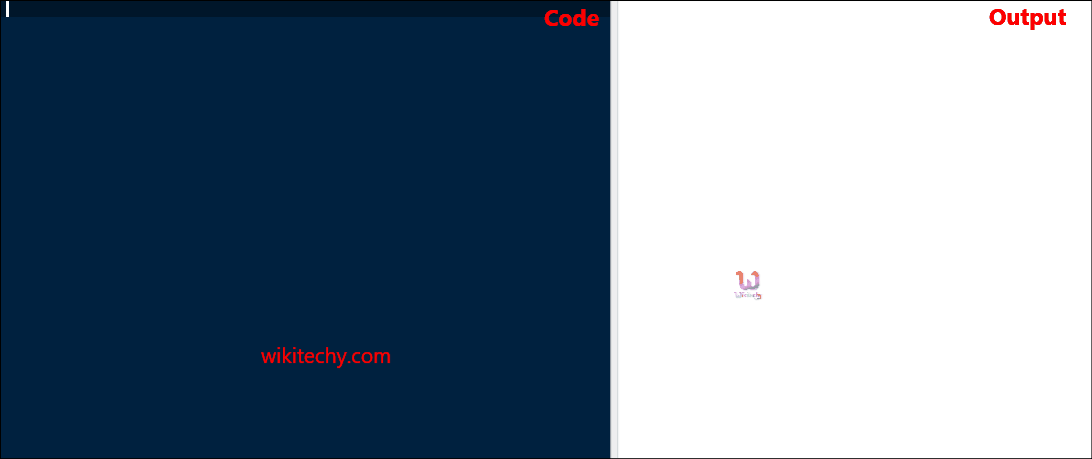
C# Foreach Loops
What is Foreach loop in C# ?
- The foreach statement is used to iterate through the collection to get the information that we want, but cannot be used to add or remove items from the source collection to avoid unpredictable side effects.
- If we need to add or remove items from the source collection, use a for loop.
- A foreach loop can also be left by the goto, return, or throw statements.
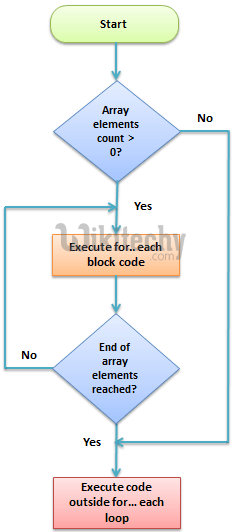
C# for each loop
Syntax:
foreach (string name in arr)
{
}
- Where, name is a string variable that takes value from collection as arr and then processes them in the body area.
C# Sample Code - C# Examples
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace wikitechy_foreach_loop
{
class Program
{
static void Main(string[] args)
{
string[] arr = new string[4];
arr[0] = "While Loop";
arr[1] = "Do While";
arr[2] = "For Loop";
arr[3] = "Foreach Loop";
foreach (string name in arr)
{
Console.WriteLine("WikiTechy says this is: " + name);
}
Console.ReadLine();
}
}
}
Code Explanation:
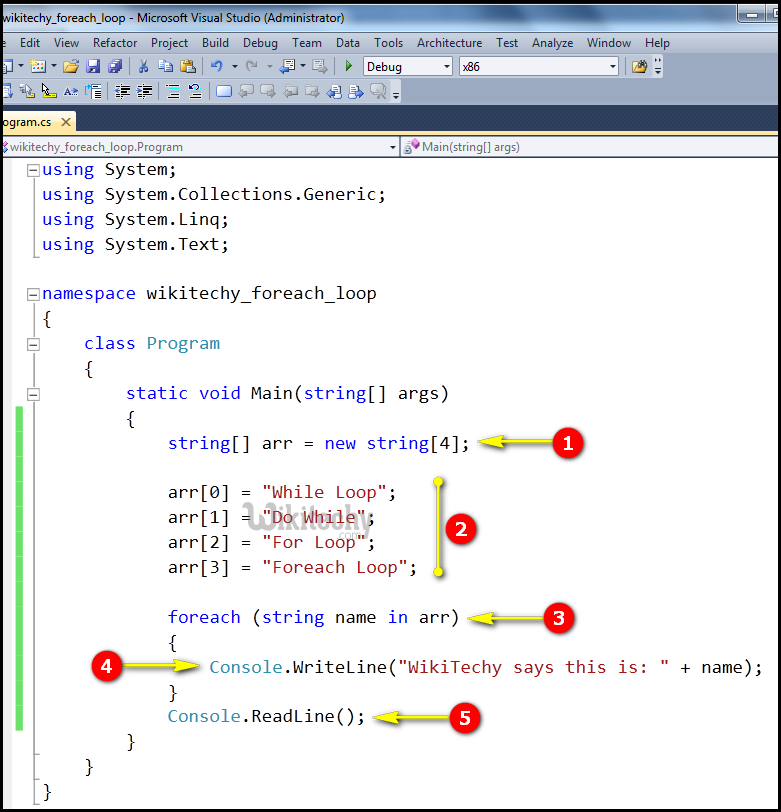
- This array contains the elements from array[0] to array[4]. The new operator is used to create the array and initialize the array elements to their default values. In this example, all the array elements are initialized to zero.
- arr[0] specifies to storing the value in array "while loop" element 1. Similarly arr[1] storing the value in array "Do while" element 2. arr[2] storing the value in array "For loop" element 3. arr[3] storing the value in array "Foreach loop" element 4.
- foreach (string name in arr) it retrieving value using foreach loop.
- In this example Console.WriteLine, the Main method specifies its behavior with the statement "WikiTtechy says this is". And WriteLine is a method of the Console class defined in the System namespace. This statement reasons the message "WikiTechy says this is" to be displayed on the screen.
- Here Console.ReadLine(); specifies to reads input from the console. When the user presses enter, it returns a string.
Sample C# examples - Output :
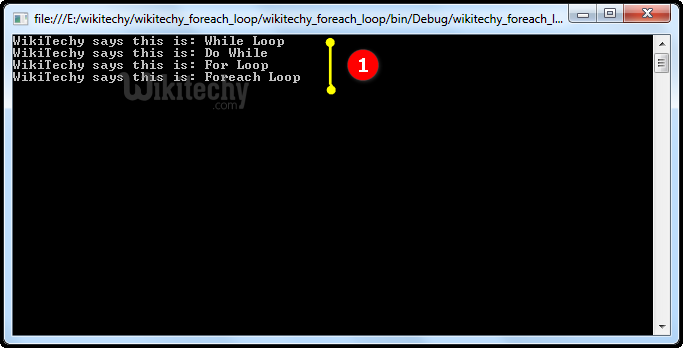
- Here in this output we display the "WikiTechy says this is : "While Loop, Do While, For Loop, Foreach Loop".