C# Namespaces - c# - c# tutorial - c# net
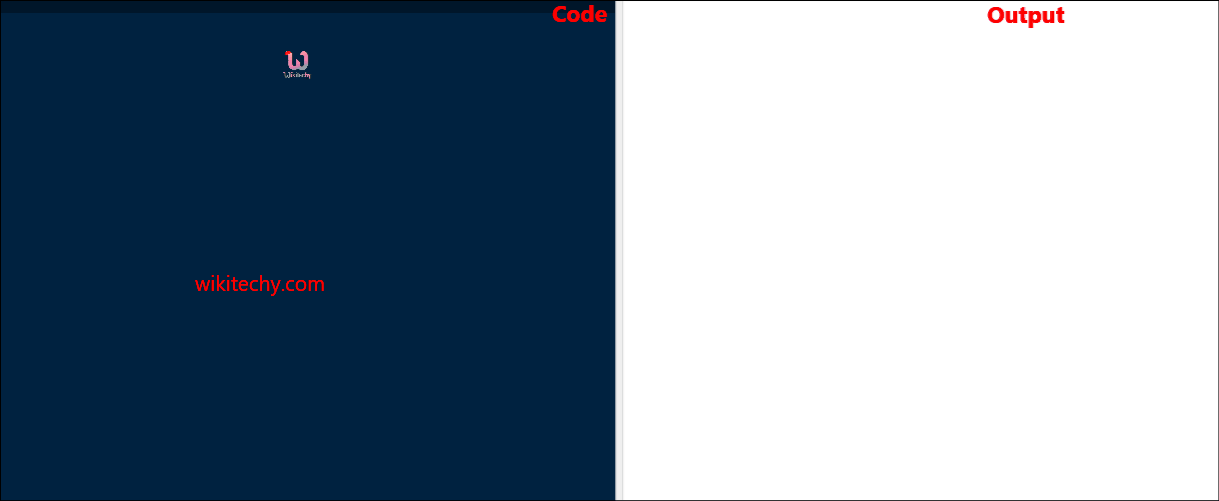
C# Namespaces
What is the use of namespace in C# ?
- Namespaces in C# are used to organize too many classes so that it can be easy to handle the application.
- In a simple C# program, we use System.Console where System is the namespace and Console is the class.
- To access the class of a namespace, we need to use namespacename.classname. We can use using keyword so that we don't have to use complete name all the time.
- In C#, global namespace is the root namespace.
- The global::System will always refer to the namespace "System" of .Net Framework.
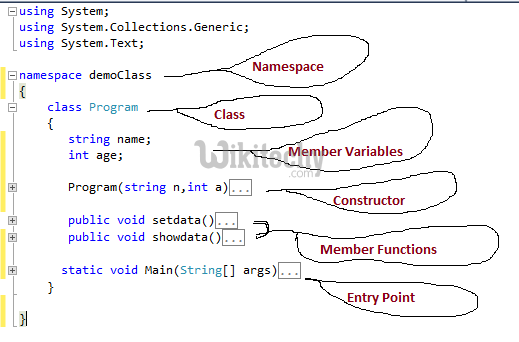
What is the use of namespace in C#
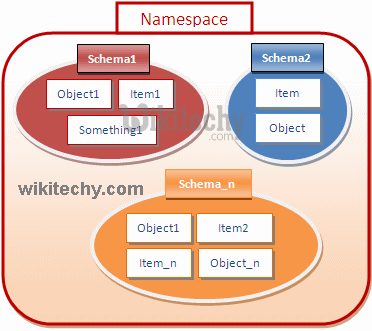
Namespace
C# namespace example
- Let's see a simple example of namespace which contains one class "Program".
using System;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello Namespace!");
}
}
}
C# examples - Output :
Hello Namespace!
C# namespace example: by fully qualified name
- Let's see another example of namespace in C# where one namespace program accesses another namespace program.
using System;
namespace First {
public class Hello
{
public void sayHello() { Console.WriteLine("Hello First Namespace"); }
}
}
namespace Second
{
public class Hello
{
public void sayHello() { Console.WriteLine("Hello Second Namespace"); }
}
}
public class TestNamespace
{
public static void Main()
{
First.Hello h1 = new First.Hello();
Second.Hello h2 = new Second.Hello();
h1.sayHello();
h2.sayHello();
}
}
C# examples - Output :
Hello First Namespace
Hello Second Namespace
C# namespace example: by using keyword
- Let's see another example of namespace where we are using "using" keyword so that we don't have to use complete name for accessing a namespace program.
using System;
using First;
using Second;
namespace First {
public class Hello
{
public void sayHello() { Console.WriteLine("Hello Namespace"); }
}
}
namespace Second
{
public class Welcome
{
public void sayWelcome() { Console.WriteLine("Welcome Namespace"); }
}
}
public class TestNamespace
{
public static void Main()
{
Hello h1 = new Hello();
Welcome w1 = new Welcome();
h1.sayHello();
w1.sayWelcome();
}
}
C# examples - Output :
Hello Namespace
Welcome Namespace