C# Binary Reader - c# - c# tutorial - c# net
What is C# BinaryReader ?
- C# BinaryReader class is used to read binary information from stream.
- It is found in System.IO namespace.
- It also supports reading string in specific encoding.
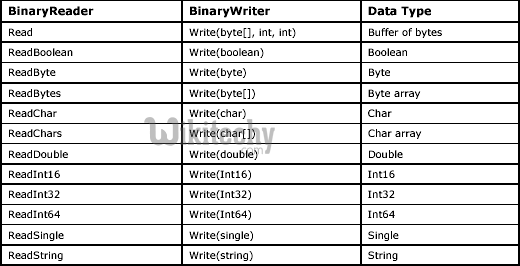
Constructors:
Name | Description |
---|---|
BinaryReader(Stream) | Initializes a new instance of the BinaryReader class based on the specified stream and using UTF-8 encoding. |
BinaryReader(Stream, Encoding) | Initializes a new instance of the BinaryReader class based on the specified stream and character encoding. |
BinaryReader(Stream, Encoding, Boolean) | Initializes a new instance of the BinaryReader class based on the specified stream and character encoding, and optionally leaves the stream open. |
Properties:
Name | Description |
---|---|
BaseStream | Exposes access to the underlying stream of the BinaryReader. |
Methods:
Name | Description |
---|---|
Close() | Closes the current reader and the underlying stream. |
Dispose() | Releases all resources used by the current instance of the BinaryReader class. |
Dispose(Boolean) | Releases the unmanaged resources used by the BinaryReader class and optionally releases the managed resources. |
Equals(Object) | Determines whether the specified object is equal to the current object.(Inherited from Object.) |
FillBuffer(Int32) | Fills the internal buffer with the specified number of bytes read from the stream. |
Finalize() | Allows an object to try to free resources and perform other cleanup operations before it is reclaimed by garbage collection.(Inherited from Object.) |
GetHashCode() | Serves as the default hash function. (Inherited from Object.) |
GetType() | Gets the Type of the current instance.(Inherited from Object.) |
MemberwiseClone() | Creates a shallow copy of the current Object.(Inherited from Object.) |
PeekChar() | Returns the next available character and does not advance the byte or character position. |
Read() | Reads characters from the underlying stream and advances the current position of the stream in accordance with the Encoding used and the specific character being read from the stream. |
Read(Byte[], Int32, Int32) | Reads the specified number of bytes from the stream, starting from a specified point in the byte array. |
Read(Char[], Int32, Int32) | Reads the specified number of characters from the stream, starting from a specified point in the character array. |
Read7BitEncodedInt() | Reads in a 32-bit integer in compressed format. |
ReadBoolean() | Reads a Boolean value from the current stream and advances the current position of the stream by one byte. |
ReadByte() | Reads the next byte from the current stream and advances the current position of the stream by one byte. |
ReadBytes(Int32) | Reads the specified number of bytes from the current stream into a byte array and advances the current position by that number of bytes. |
ReadChar() | Reads the next character from the current stream and advances the current position of the stream in accordance with the Encoding used and the specific character being read from the stream. |
Read Also
.net course online free , dot net training institute near me , dot net developer internshipC# BinaryReader Example:
- Let's see the simple example of BinaryReader class which reads data from data file.
using System;
using System.IO;
namespace BinaryWriterExample
{
class Program
{
static void Main(string[] args)
{
WriteBinaryFile();
ReadBinaryFile();
Console.ReadKey();
}
static void WriteBinaryFile()
{
using (BinaryWriter writer = new BinaryWriter(File.Open("e:\\binaryfile.dat", FileMode.Create)))
{
writer.Write(12.5);
writer.Write("this is string data");
writer.Write(true);
}
}
static void ReadBinaryFile()
{
using (BinaryReader reader = new BinaryReader(File.Open("e:\\binaryfile.dat", FileMode.Open)))
{
Console.WriteLine("Double Value : " + reader.ReadDouble());
Console.WriteLine("String Value : " + reader.ReadString());
Console.WriteLine("Boolean Value : " + reader.ReadBoolean());
}
}
}
}
C# examples - Output :
Double Value : 12.5
String Value : this is string data
Boolean Value : true