C# try catch finally - c# - c# tutorial - c# net
What is the use of try-catch-finaly statement in C# ?
- C# finally block is used to execute important code which is to be executed whether exception is handled or not. It must be preceded by catch or try block.
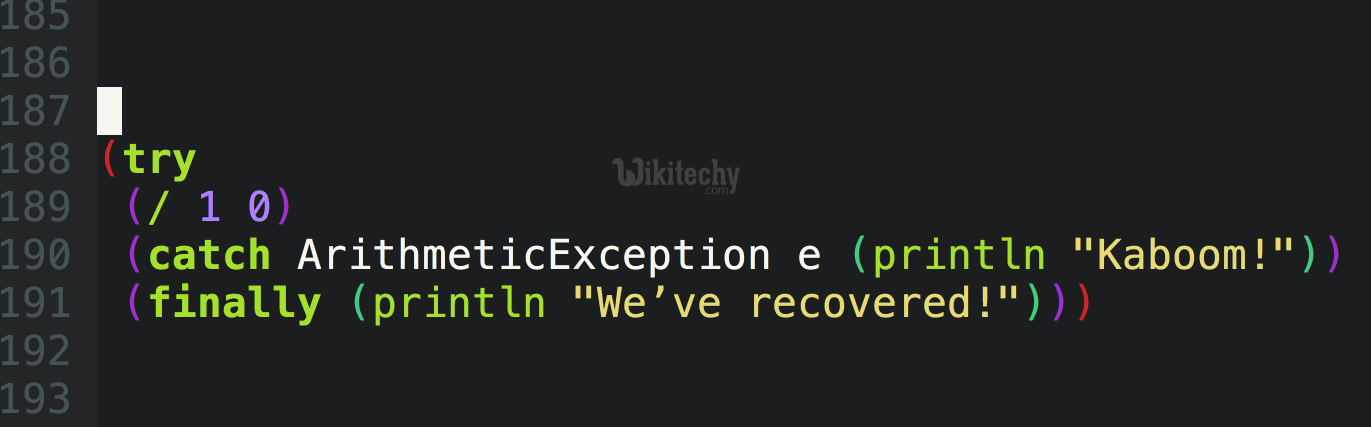
Try Catch Finally Exception
C# finally example if exception is handled
using System;
public class ExExample
{
public static void Main(string[] args)
{
try
{
int a = 10;
int b = 0;
int x = a / b;
}
catch (Exception e) { Console.WriteLine(e); }
finally { Console.WriteLine("Finally block is executed"); }
Console.WriteLine("Rest of the code");
}
}
C# examples - Output :
System.DivideByZeroException: Attempted to divide by zero.
Finally block is executed
Rest of the code
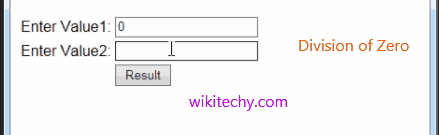
Exception Handling
C# finally example if exception is not handled
using System;
public class ExExample
{
public static void Main(string[] args)
{
try
{
int a = 10;
int b = 0;
int x = a / b;
}
catch (NullReferenceException e) { Console.WriteLine(e);
}
finally { Console.WriteLine("Finally block is executed"); }
Console.WriteLine("Rest of the code");
}
}
C# examples - Output :
Unhandled Exception: System.DivideBy