Java vs C# - c# - c# tutorial - c# net
What is the difference between Java and C# ?
- Java is an Object-Oriented, general-purpose programming language and class-based. Developers can use the principle – “write once, run anywhere” with Java.
- Java source program is converted to bytecode by the Java compiler, and then this compiled bytecode can be executed on any operating system having compatible JRE (Java Runtime Environment).
- C# is an Object-Oriented, functional, generic, and component-oriented programming language.
- C# is used to build various applications; it is specifically strong at building Windows desktop applications and games. Web development can also be done efficiently with C#, which has increasingly become popular for mobile development.
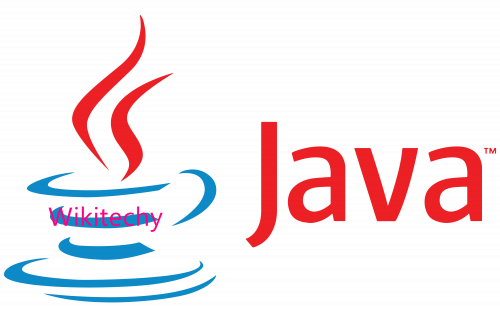
What is Java ?
- James Gosling originally developed Java at Sun Microsystem.C++, another class-based and object-oriented programming language, largely influences Java’s syntax. The JDK (Java Development Kit) contains several components required to run a Java program, although not all components of JDK are necessary to run Java. The latest version of Java is JDK 21, released in September 2023.
- Once written in Java, the source code can be run on any platform, which is one of the most significant benefits. Java programs can run on any hardware and operating system combination because Java is a portable language.
- To run a Java program, one must install an appropriate JRE (Java Runtime Environment) on the required operating system, which is available for download on Java’s official website.
- A compiler would convert Java source code to bytecode, and JVM (Java Virtual Machine), created inside JRE (Java Runtime Environment), would convert bytecode to machine code. Java has extensive support for concurrency, networking, and GUI (Graphic User Interface).
Sample Code
class Program
{
public static void main(String[ ] args)
{
int a = 10;
int b = 2;
int c = a * b;
System.out.print("The result is " + c);
}
}
Output
The result is 20
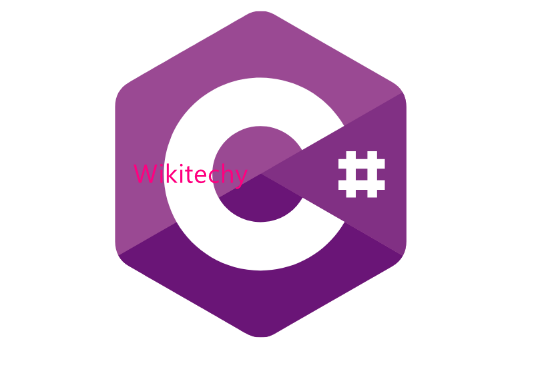
What is C# ?
- C# was developed by Microsoft with its .NET initiative, with a development team led by Anders Hejlsberg. The latest version of C# is 11.02, released in 2022, along with visual studio 2022 version 17.7 So, it is an excellent choice for any programmer who wishes to develop web and games.
- The language abstracts away many complex tasks, so developers do not have to worry about issues like memory management and garbage collection while developing logic for an application or game. Additionally, C# is a high-level language that is easier to read than other programming languages.
- C# checks the written source code before it becomes an application, as it is a statically-typed language. C# is a complex language to learn, and mastering it can take more time than a language like Python. One who wishes to build advanced programs with C# must know a substantial amount of code. It has proliferated since it was first created, with extensive support from Microsoft.
Sample Code
class Program
{
public static void Main(String[ ] args)
{
int a = 10;
int b = 2;
int c = a * b;
Console.WriteLine("The result is " + c);
}
}
Output
The result is 20
When were Java and C# first released?
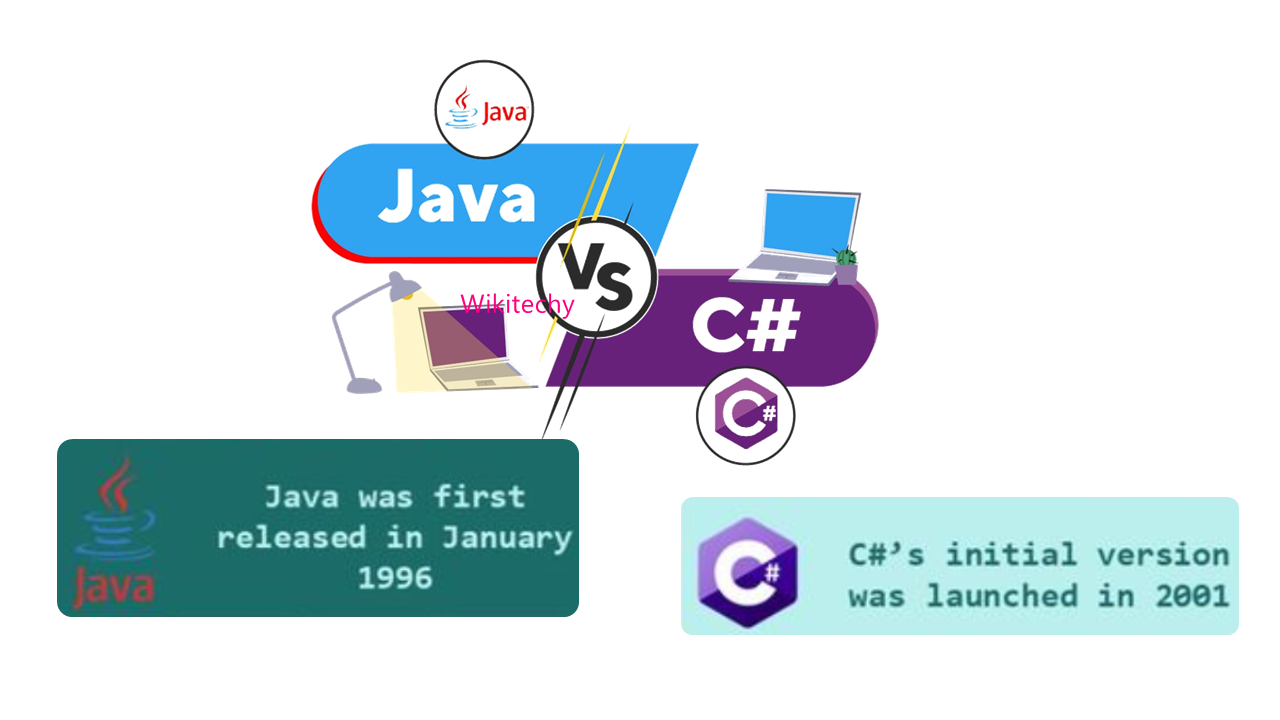
- Java: Java's first official version, Java 1.0, was released by Sun Microsystems on January 23, 1996.
- C#: C# 1.0 was released by Microsoft as a part of the .NET Framework on December 2001.
Who are the authors of Java and C#?
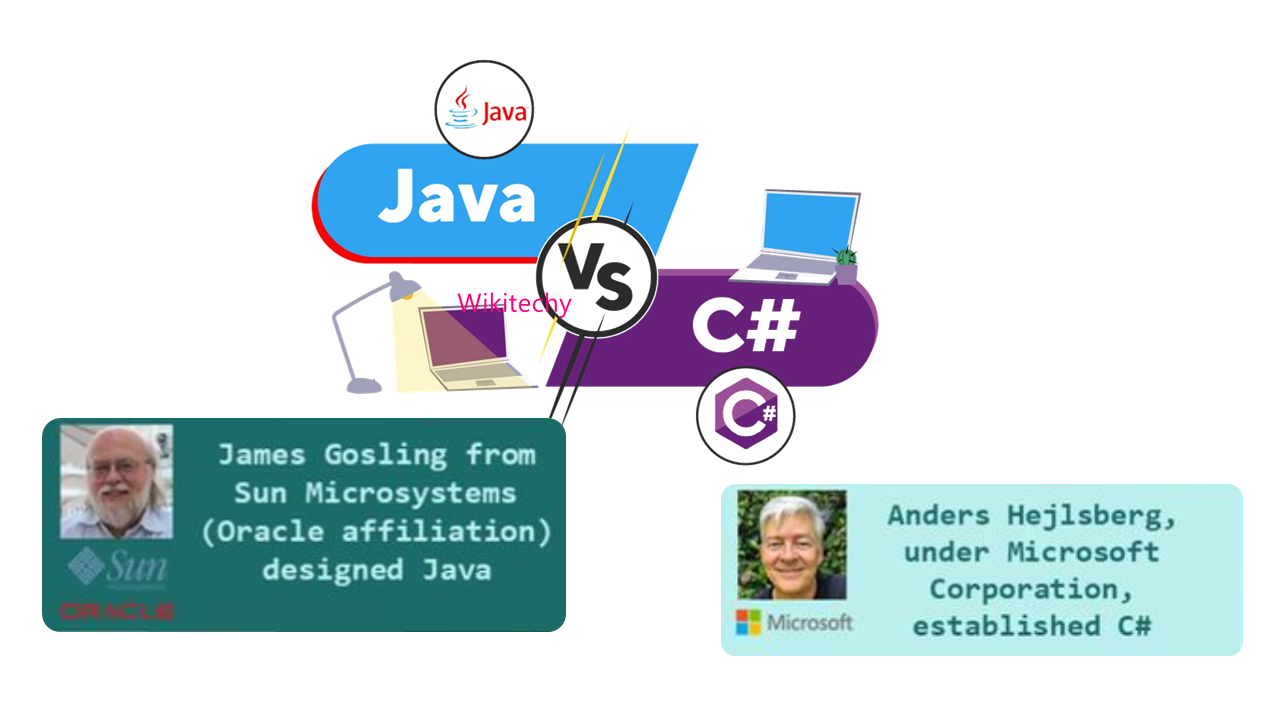
- Java: Java was developed by James Gosling, Mike Sheridan, and Patrick Naughton at Sun Microsystems. It was officially released by Sun Microsystems in 1996.
- C#: C# was developed by Anders Hejlsberg and his team at Microsoft. It was officially released by Microsoft as part of the .NET Framework in 2001. Anders Hejlsberg is widely recognized as the lead architect of C#.
Java and C# Code Architecture
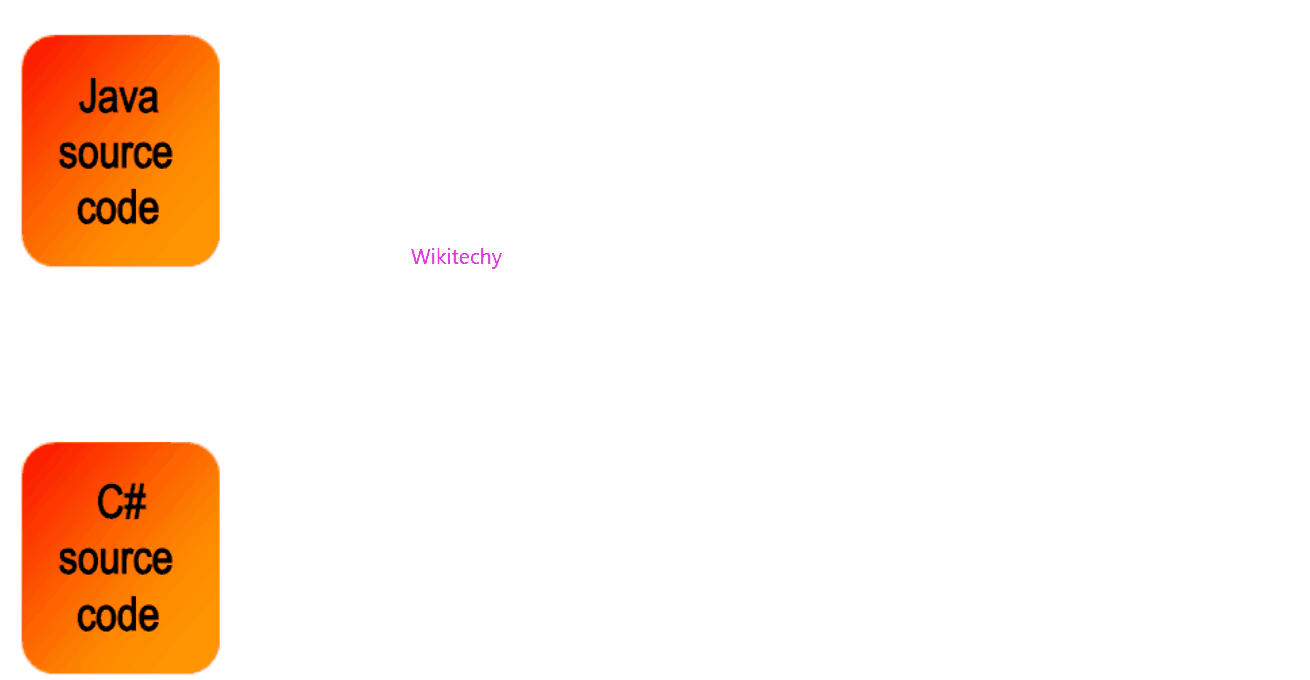
For Java:
- Java Source Code (.java):
- Java programs start as human-readable source code files with a .java extension.
- Compilation to Java Bytecode (.class):
- The Java compiler (javac) compiles the .java source files into Java bytecode files (.class files). Java bytecode is platform-independent and can be executed on any Java Virtual Machine (JVM).
- Java Bytecode to JAR file (.jar):
- Java bytecode files can be bundled into JAR (Java Archive) files. A JAR file is a package file format used to aggregate many Java class files, associated metadata, and resources into one file.
For C#:
- C# Source Code (.cs):
- C# programs begin as human-readable source code files with a .cs extension.
- Compilation to Common Intermediate Language (CIL):
- The C# compiler (csc or dotnet build) compiles the .cs source files into an intermediate language called Common Intermediate Language (CIL) code. CIL is platform-independent and can run on any Common Language Runtime (CLR).
- CIL to .NET Module File (.module):
- CIL code can be compiled into a .NET module file (.module) if you want to create a reusable module. A module file can contain CIL code, metadata, and resources.
- .NET Module to DLL File (.dll):
- The module file can be assembled into a Dynamic Link Library (DLL) file. A DLL file is a compiled library that contains code, data, and resources used by .NET applications. DLLs are executable files used for deploying and sharing libraries of code.
Java Compiler
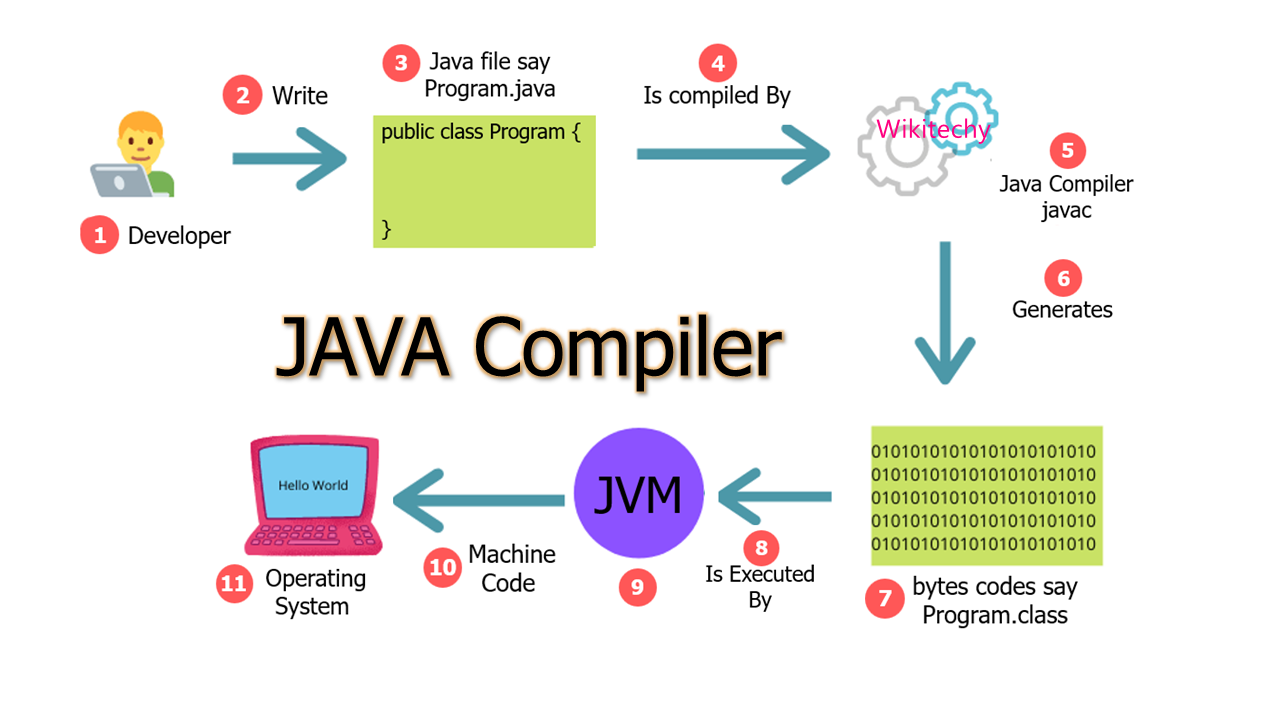
1. Developer Writes Java Code:
- A developer writes Java source code in a file, typically with a .java extension. For example, let's call the file program.java.
2. Compilation using Java Compiler (javac):
- The developer uses the Java compiler (javac), a part of the Java Development Kit (JDK), to compile the Java source file (program.java).
- The Java compiler checks the source code for syntax errors and compiles it into bytecode. If there are no syntax errors, it generates bytecode files. Bytecode is a platform-independent representation of the program, stored in files with a .class extension. In this case, it would generate program.class.
3. Generated Bytecode (program.class):
- The program.class file contains bytecode instructions that are understandable by the Java Virtual Machine (JVM). Bytecode is a low-level representation of the source code, which is designed to be executed by the JVM.
4. Execution by Java Virtual Machine (JVM):
- To run the Java program, the developer uses the Java interpreter, which is a part of the Java Runtime Environment (JRE). The interpreter loads and executes the bytecode generated by the compiler.
- The JVM interprets the bytecode instructions and executes them, performing the operations specified in the Java source code.
5. Conversion to Machine Code:
- During execution, the JVM's Just-In-Time (JIT) compiler may convert frequently executed bytecode into native machine code. This compilation happens at runtime and is specific to the underlying hardware and operating system, optimizing the program's performance.
6. Execution by the Operating System:
- The converted machine code is executed directly by the computer's hardware, as directed by the operating system.
So, in summary:
- Developer writes Java code (program.java) -> Compilation (javac) -> Bytecode (program.class) -> Execution by JVM -> Compilation to Machine Code (JIT) -> Execution by the Operating System
- This process enables Java programs to be platform-independent, as they are compiled to bytecode that can run on any system with a compatible Java Virtual Machine.
C# Compiler
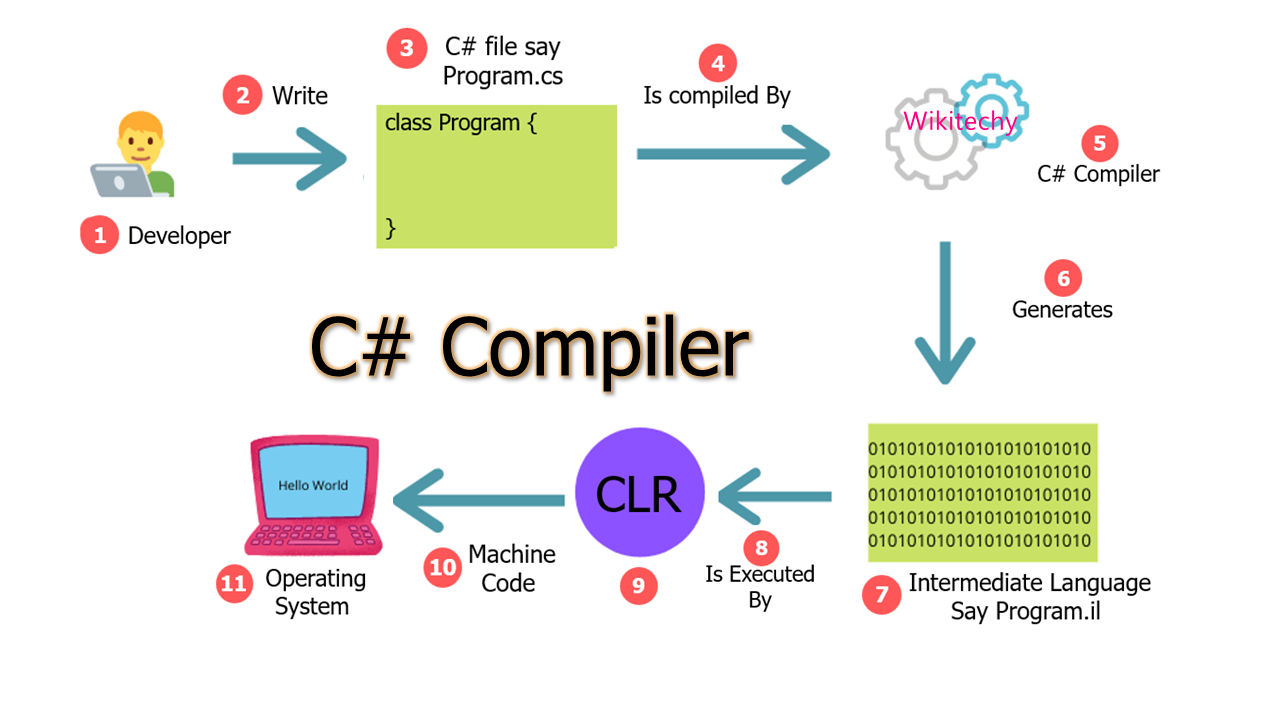
1. Developer Writes C# Code:
- The process begins with a developer writing C# source code in a text file. The source code typically has a .cs file extension. For example, let's say the developer creates a file named program.cs and writes C# code in it.
2. Compilation with C# Compiler:
- To transform the human-readable C# source code into a format that the computer can understand, the developer uses the C# Compiler, which is usually invoked from the command line with the csc (C# Compiler) command. The C# Compiler reads the program.cs file and checks it for syntax errors and compliance with the C# language specifications.
3. Generation of Intermediate Language (IL) Code (.il):
- If the C# Compiler doesn't find any errors, it generates Intermediate Language (IL) code. IL code is a low-level, platform-independent representation of the C# source code. The compiler creates one or more files with a .il file extension, e.g., program.il. These .il files contain instructions that a Common Language Runtime (CLR) can execute.
4. Execution by the Common Language Runtime (CLR):
- The IL code is not machine code but rather a set of instructions that are executed by the Common Language Runtime (CLR). The CLR is a component of the .NET Framework responsible for managing the execution of .NET programs. It takes the IL code from program.il and performs Just-In-Time (JIT) compilation, translating the IL code into machine code that can be executed by the host operating system.
5. Machine Code Execution:
- The JIT compiler translates the IL code into machine code that is specific to the underlying hardware and operating system. This machine code is executed directly by the host operating system on the computer's processor.
So, in summary:
- Developer writes C# code (program.cs).
- C# Compiler (csc) compiles the source code into Intermediate Language (IL) code (program.il).
- Common Language Runtime (CLR) executes the IL code and translates it into machine code using Just-In-Time (JIT) compilation.
- The machine code is executed by the host operating system.
This process allows C# applications to be platform-independent at the source code level, as long as a compatible CLR is available for the target platform. The CLR takes care of adapting the IL code to the specific hardware and operating system where the C# program is run.
Java vs C# Cross Platform
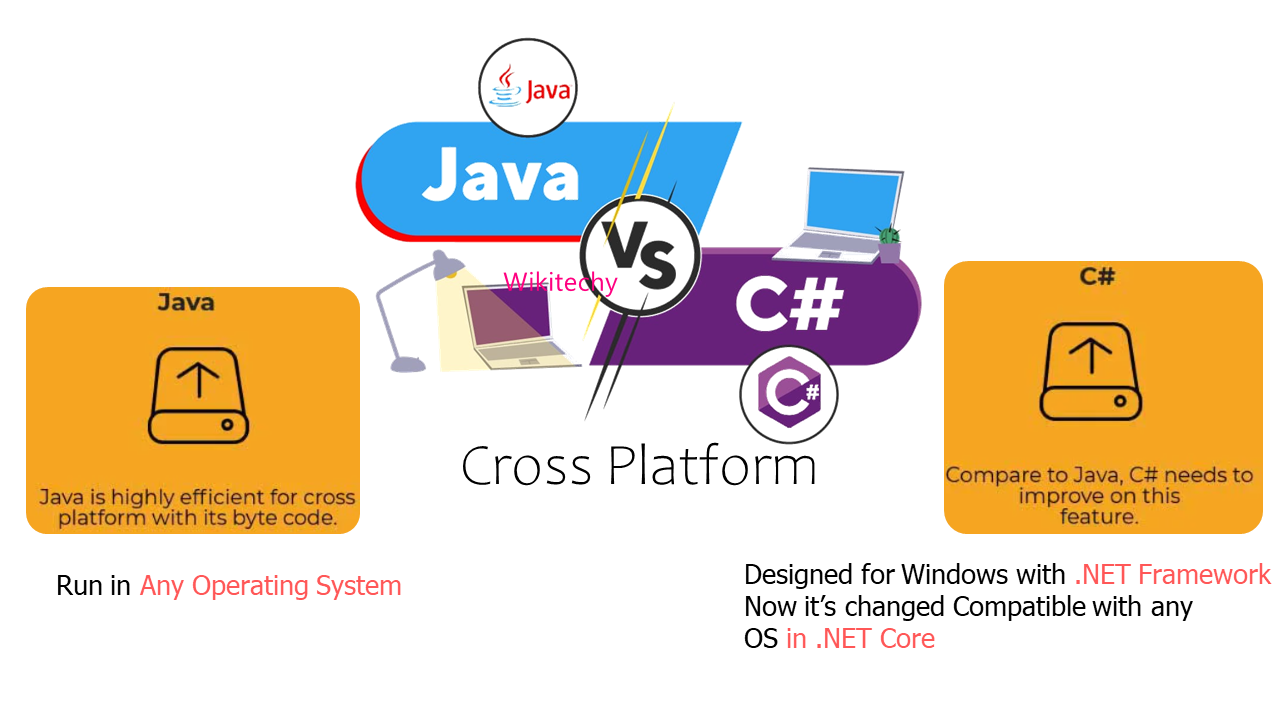
Both Java and C# are designed to be cross-platform, meaning that you can write code once and run it on different operating systems without modification, as long as the necessary runtime environments are available on those platforms.
1. Java:
- Write Once, Run Anywhere (WORA): Java is famous for its platform independence due to the Java Virtual Machine (JVM). Java code is compiled into bytecode, which is executed by the JVM. As long as there is a JVM available for an operating system, Java applications can run on that OS without modification.
- Operating System Independence: Java applications can run on Windows, Linux, macOS, and various other platforms with a compatible JVM installed.
2.C#:
- Cross-Platform Execution with .NET Core/.NET 5+: While C# originated as a Microsoft language, the introduction of .NET Core (which evolved into .NET 5 and beyond) made it cross-platform. .NET Core is a free, open-source, and cross-platform framework that supports C# development on Windows, Linux, and macOS.
- ASP.NET Core for Web Applications: ASP.NET Core, a framework for building web applications, is fully cross-platform and can be hosted on different operating systems.
- Xamarin for Mobile Applications: Xamarin, a framework based on .NET, allows developers to create cross-platform mobile applications for iOS and Android using C#.
3. Development Tools:
- Java: Java development can be done using IDEs like Eclipse, IntelliJ IDEA, or NetBeans, which are available on multiple platforms.
- C#: C# development can be done using Visual Studio, which is available on Windows and macOS. Visual Studio Code, a lightweight and cross-platform code editor, also supports C# development.
Java vs C# Applications
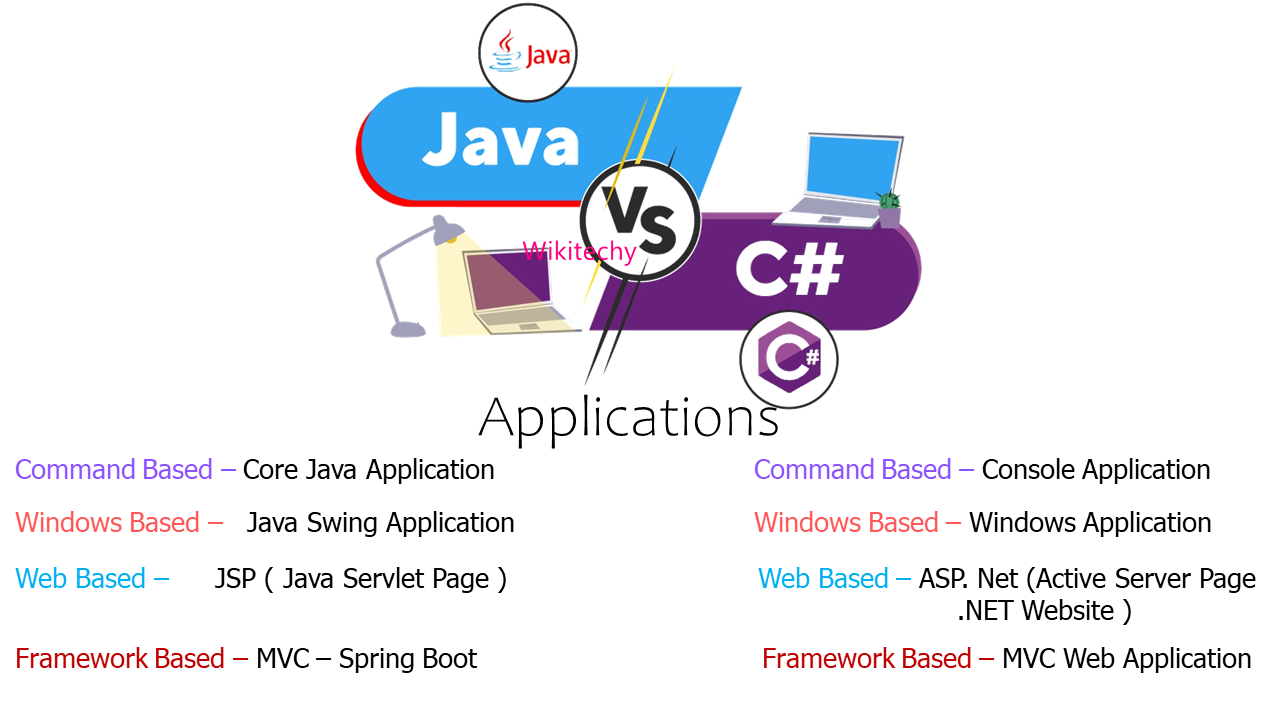
1. Command-Based – Core Java Application vs. Command-Based – Console Application:
- Java: Core Java Application refers to a basic Java program without any graphical user interface.
- .NET: Console Application refers to a .NET application that runs in a console window and interacts via text input/output.
2. Windows-Based – Java Swing Application vs. Windows-Based – Windows Application:
- Java: Java Swing Application is a Java-based graphical user interface application for desktop platforms.
- .NET: Windows Application typically refers to a Windows Forms Application in the .NET framework, providing a GUI for Windows-based systems.
3. Web-Based – JSP (Java Servlet Page) vs. Web-Based – ASP.NET (Active Server Page .NET Website):
- Java: JSP (Java Servlet Page) is a technology used for creating dynamic web pages in Java, often used with servlets.
- .NET: ASP.NET is a web development framework from Microsoft, allowing developers to build dynamic web applications and websites using .NET technologies.
4. Framework-Based – MVC – Spring Boot vs. Framework-Based – .NET MVC Web Application:
- Java: Spring Boot is a Java-based framework that simplifies the development of production-ready applications, including MVC web applications following the Spring MVC pattern.
- .NET: .NET MVC (Model-View-Controller) is a framework in the Microsoft .NET ecosystem, allowing developers to build web applications following the MVC architectural pattern.
Each of these pairs represents different technologies or frameworks for building similar types of applications in the Java and .NET ecosystems. The choice between them often depends on factors such as developer expertise, project requirements, and the specific features offered by each technology.
A list of top differences between Java and C# are given below:
No. | Java | C# |
---|---|---|
1) Java Vs C# | Java is a high level, robust, secured and object-oriented programming language developed by Oracle. |
C# is an object-oriented programming language developed by Microsoft that runs on .Net Framework. |
2) Platform and Runtimes | Java programming language is designed to be run on a Java platform, by the help of Java Runtime Environment (JRE). |
C# programming language is designed to be run on the Common Language Runtime (CLR). |
3) Type Safety | Java type safety is safe. | C# type safety is unsafe. |
4) Data Types | In java, built-in data types that are passed by value are called primitive types. |
n C#, built-in data types that are passed by value are called simple types. |
5) Arrays | Arrays in Java are direct specialization of Object. | Arrays in C# are specialization of System. |
6) Conditional Compilation | Java does not support conditional compilation. | C# supports conditional compilation using preprocessor directives |
7) Goto Statement | Java doesn't support goto statement. | C# supports goto statement. |
8) Inheritance | java doesn't support multiple inheritance through class. It can be achieved by interfaces in java |
C# supports multiple inheritance using class. |
9) Structure and Unions | Java doesn't support structures and unions. | C# supports structures and unions. |
10) Exception Handling | Java supports checked exception and unchecked exception. |
C# supports unchecked exception. |
11) Paradigm | Class-based, an Object-Oriented language derived from C++ | Object-Oriented, component-oriented, functional, strong typing |
12) Application | Complex web-based, highly concurrent application | Web and game development, popular for mobile development |
13) Project | Suited for complex web-based concurrency project | Best suited for game development projects |
14) Usage | Messaging, web application, highly concurrent application | Games, mobile development, virtual reality |
15) Installation | Require JDK (Java Development Kit) to run Java | .NET framework provides a huge library of codes used by C# |
16) Scope | Dominate server-side interaction | Server-side language with a good programming foundation |
17) Cross-platform | Java is highly efficient for cross-platform with its bytecode | Compared to Java, C# needs to improve on this feature |
18) Tools | Eclipse, NetBeans, IntelliJ IDEA | Visual Studio, MonoDevelop, #develop |