C# inheritance | inheritance in c# | inheritance c# - c# - c# tutorial - c# net
What is inheritance in C# ?
- In C#, inheritance is a process in which one object acquires all the properties and behaviors of its parent object automatically.
- In such way, you can reuse, extend or modify the attributes and behaviors which is defined in other class.
- In C#, the class which inherits the members of another class is called derived class and the class whose members are inherited is called base class.
- The derived class is the specialized class for the base class.
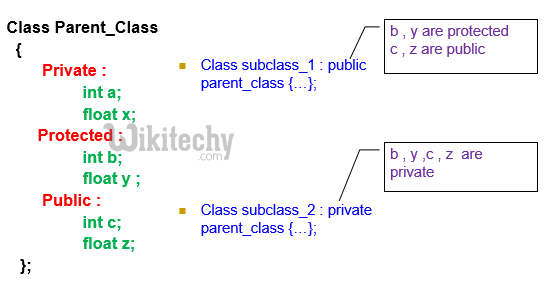
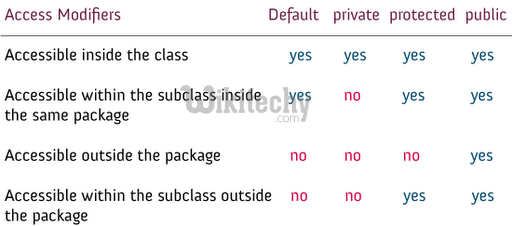
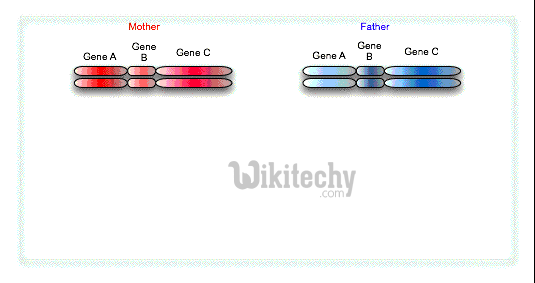
Inheritance
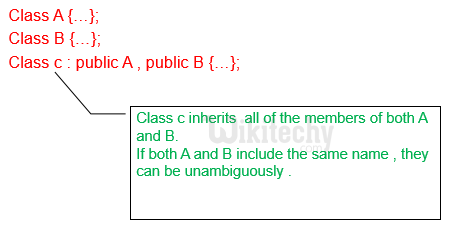
Advantage of C# Inheritance
Code reusability:
- Now you can reuse the members of your parent class. So, there is no need to define the member again. So less code is required in the class.
C# Single Level Inheritance Example: Inheriting Fields
- When one class inherits another class, it is known as single level inheritance.
- Let's see the example of single level inheritance which inherits the fields only.
using System;
public class Employee
{
public float salary = 40000;
}
public class Programmer: Employee
{
public float bonus = 10000;
}
class TestInheritance{
public static void Main(string[] args)
{
Programmer p1 = new Programmer();
Console.WriteLine("Salary: " + p1.salary);
Console.WriteLine("Bonus: " + p1.bonus);
}
}
C# examples - Output :
Salary: 40000
Bonus: 10000
- In the above example, Employee is the base class and Programmer is the derived class.
C# Single Level Inheritance Example: Inheriting Methods
- Let's see another example of inheritance in C# which inherits methods only.
using System;
public class Animal
{
public void eat() { Console.WriteLine("Eating..."); }
}
public class Dog: Animal
{
public void bark() { Console.WriteLine("Barking..."); }
}
class TestInheritance2{
public static void Main(string[] args)
{
Dog d1 = new Dog();
d1.eat();
d1.bark();
}
}
C# examples - Output :
Eating...
Barking...
C# Multi Level Inheritance Example
- When one class inherits another class which is further inherited by another class, it is known as multi level inheritance in C#.
- Inheritance is transitive so the last derived class acquires all the members of all its base classes.
- Let's see the example of multi level inheritance in C#.
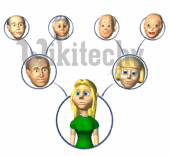
c# Inheritance
using System;
public class Animal
{
public void eat() { Console.WriteLine("Eating..."); }
}
public class Dog: Animal
{
public void bark() { Console.WriteLine("Barking..."); }
}
public class BabyDog : Dog
{
public void weep() { Console.WriteLine("Weeping..."); }
}
class TestInheritance2{
public static void Main(string[] args)
{
BabyDog d1 = new BabyDog();
d1.eat();
d1.bark();
d1.weep();
}
}
Read Also
dotnet institute in chennai , .net internship near me , .net training institute in chennaiC# examples - Output :
Eating...
Barking...
Weeping...
c# public protected private access specifiers :
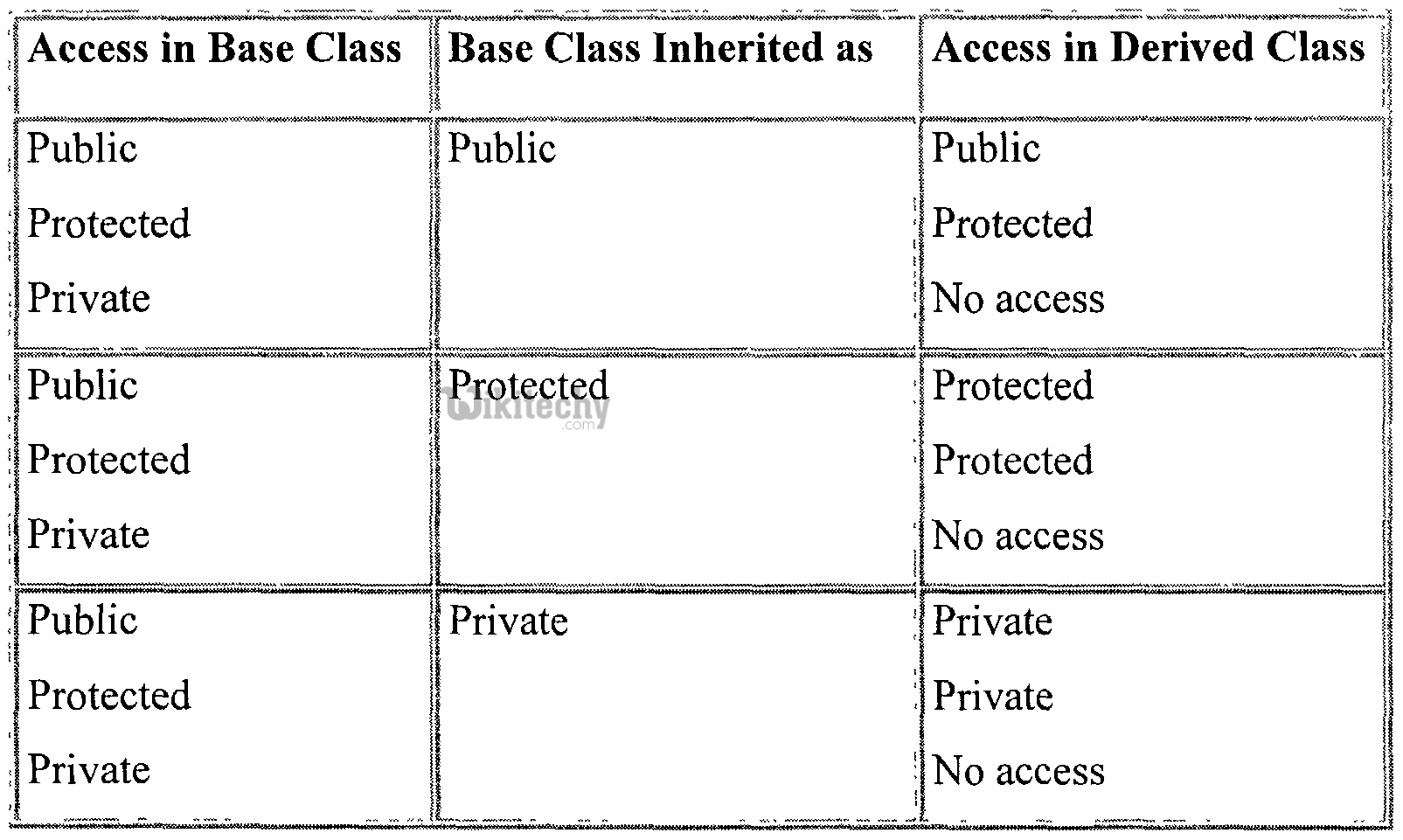