C# For Loop - c# - c# tutorial - c# net
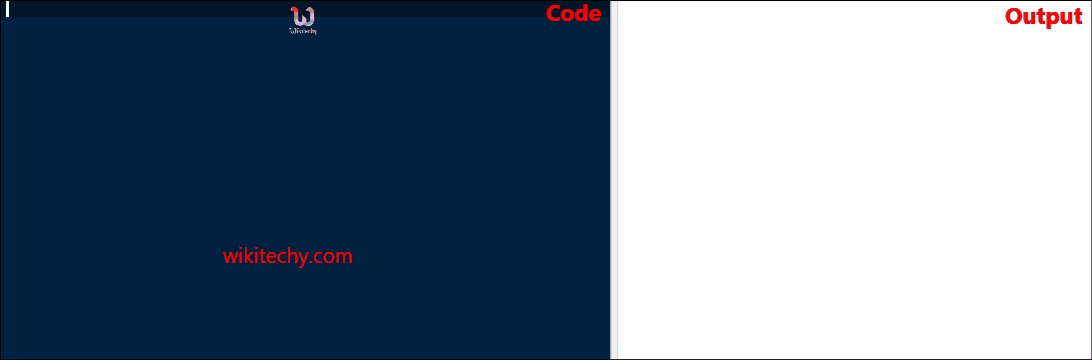
C# For Loop
What is for loop in C# ?
- For loop statement is executes a sequence of statements multiple times and abbreviates the code that manages the loop variable.
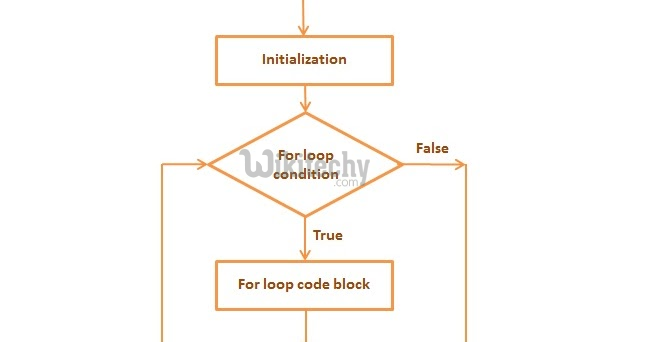
Syntax:
for(initialization counter; test counter; increment counter)
{
code to be executed;
}
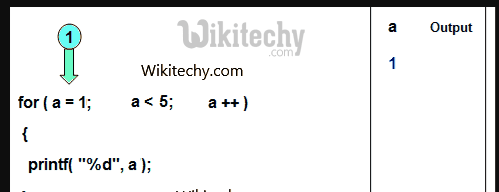
c sharp for loop
Syntax Explanation:
- initialization: It is used to initialize the counter variables.
- test counter: Evaluate for each loop iteration. If it evaluates to be TRUE, the loop continues. If it evaluates to be FALSE, the loop ends.
- increment: It increase the loopcounter with a new value. It is evaluating at the end of each iteration.
- execute the statement: It executes the statements.
Possible for loops :
for (int counter = 0, val1 = 10; counter < val1; counter++)
for ( ; counter < 100; counter+=10) // No initialization
for (int j = 0; ; j++) // No conditional expression
for ( ; j < 10; counter++, j += 3) // Compound update
for (int aNum = 0; aNum < 101; sum += aNum, aNum++) // Null loop body
;
for (int j = 0,k = 10; j < 10 && k > 0; counter++, j += 3)
C# Sample Code - C# Examples:
using System;
usingSystem.Collections.Generic;
usingSystem.Linq;
usingSystem.Text;
namespace wikitechy_for_loop
{
classProgram
{
staticvoid Main(string[] args)
{
for (int n = 5; n <= 10; n = n + 1)
{
Console.WriteLine("value of n is : {0}", n);
}
Console.ReadLine();
}
}
}
Code Explanation:
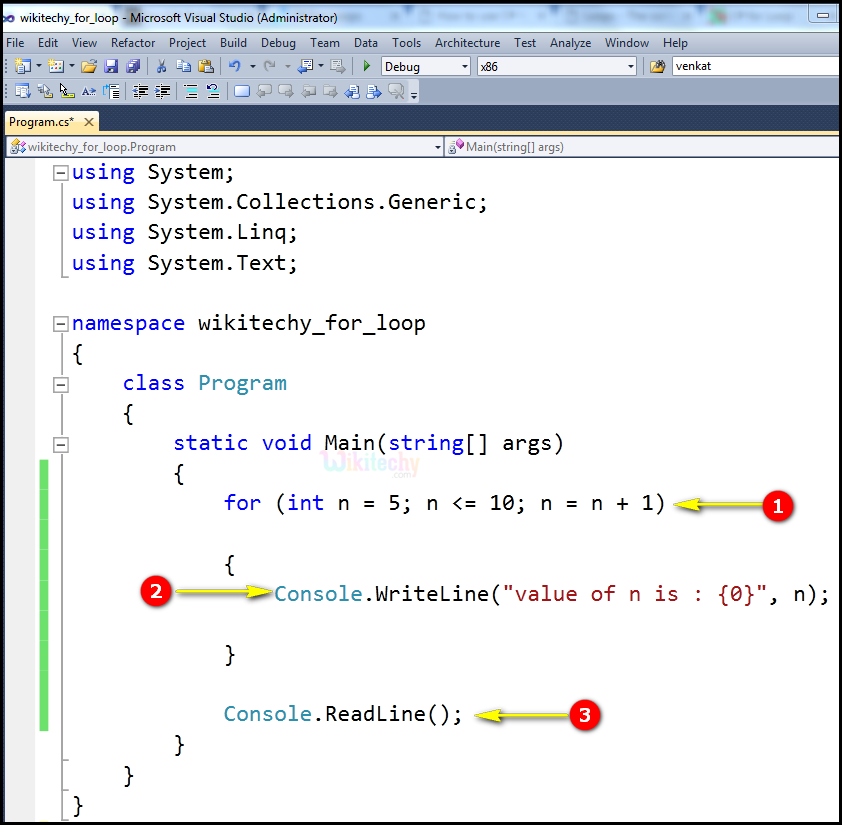
- Here for (int n = 5; n <= 10; n=n + 1)int n=5 is an integer variable. which means the expression, (n>=10),conditionis true. Therefore, the conslole.writeLine namespace statement is executed, and n=n+1 gets incremented by 1 and becomes 10.
- In this example Console.WriteLine,the Main method specifies its behavior with the statement "value of n is: {0}".AndWriteLine is a method of the Console class defined in the System namespace. This statement reasons the message""value of n is: {0}"to be displayed on the screen.
- Here Console.ReadLine(); specifies to reads input from the console. When the user presses enter, it returns a string.
Sample C# examples - Output :
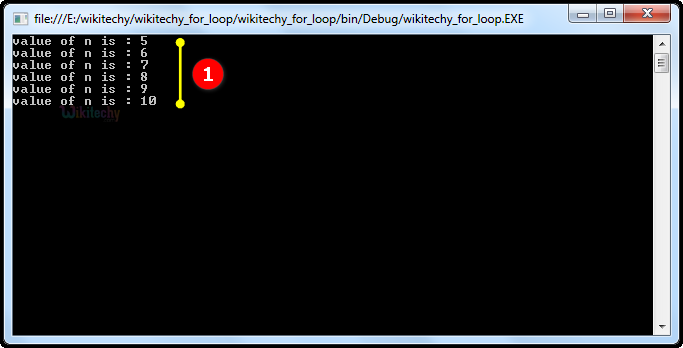
- Here in this output the value of n is:5,6,7,8,9,10 will be printed until it reaches the last element "10".