C# Checkbox | C# Controls Datagridview Add Checkbox Column - c# - c# tutorial - c# net
What is Datagridview ?
- The DataGridView control is designed to be a complete solution for displaying tabular data with Windows Forms.
- The DataGridView control is highly configurable and extensible, and it provides many properties, methods, and events to customize its appearance and behavior.
- The DataGridView control provides TextBox, CheckBox, Image, Button, ComboBox and Link columns with the corresponding cell types.
Add Checkbox Column
- In general, adding a CheckBox in a cell, the cell Value property can be set explicitly through programmatic code that accesses the cell in the Cells collection of the row, or it can be set through data binding.
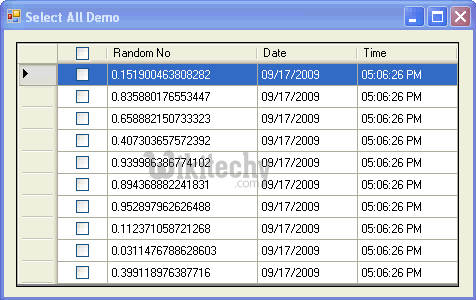
Gridview Checkbox
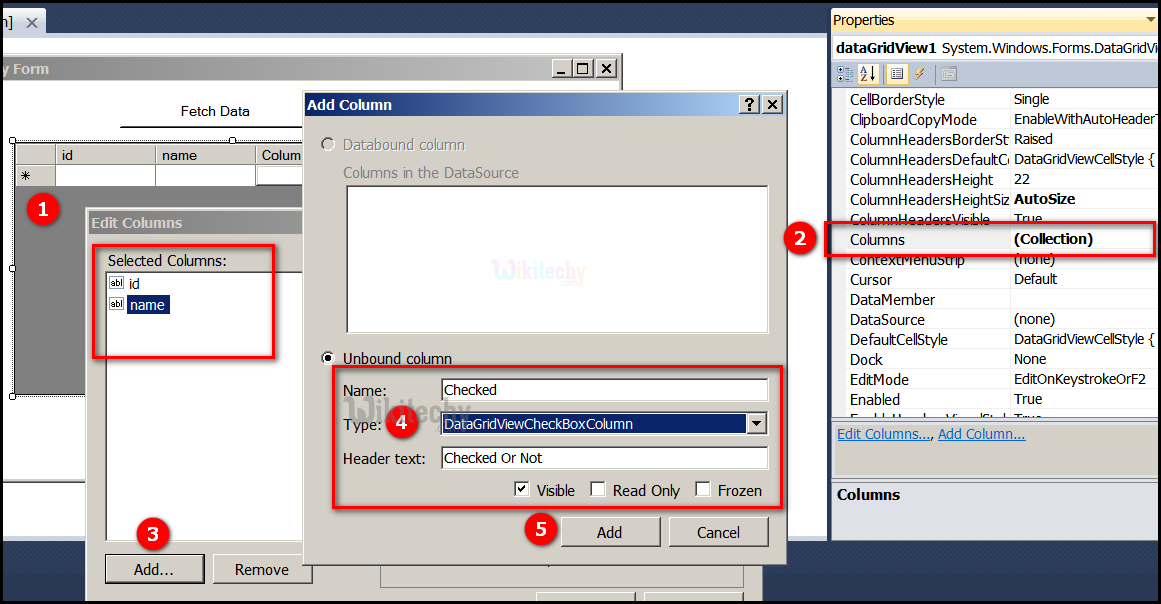
- Go to tool box and click on the DataGridview option the form will be open.
- Go to properties select Columns option and then click on the Collections, the String Collection Editor window will be open pop up where we can type strings. column collection is representing a collection of DataColumn objects for a DataTable.
- Click on "Add" button another Add column windows form will be open.
- After click on text box, and then select the DataGrideViewImage Column.
- And then click on the "Add" button, "Image" name is adding in Selected Columns windows form.
Sample C# examples - Output :
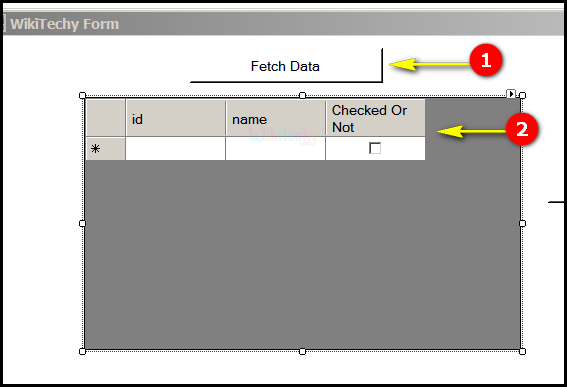
- Here in this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Here in this output table displays the Image, id, Checked Or Not.
C# Sample Code - C# Examples
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace WikiTechy_Csharp_Windows_Application
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
DataSet ds = new DataSet();
private void button1_Click(object sender, EventArgs e)
{
string connectionString = @"Data Source=VENKAT\KAASHIV;Initial Catalog=wikitechy;
user id = venkat;password=venkat";
string sql = "SELECT * FROM login_table";
SqlConnection connection = new SqlConnection(connectionString);
SqlDataAdapter dataadapter = new SqlDataAdapter(sql, connection);
connection.Open();
dataadapter.Fill(ds);
connection.Close();
dataGridView1.DataSource = ds.Tables[0];
}
private void dataGridView1_CellClick(object sender, DataGridViewCellEventArgs e)
{
if (e.ColumnIndex == 0)
{
MessageBox.Show((e.RowIndex + 1) + " Row " + (e.ColumnIndex + 1) +
" Column check box clicked ");
}
}
}
}
Code Explanation:
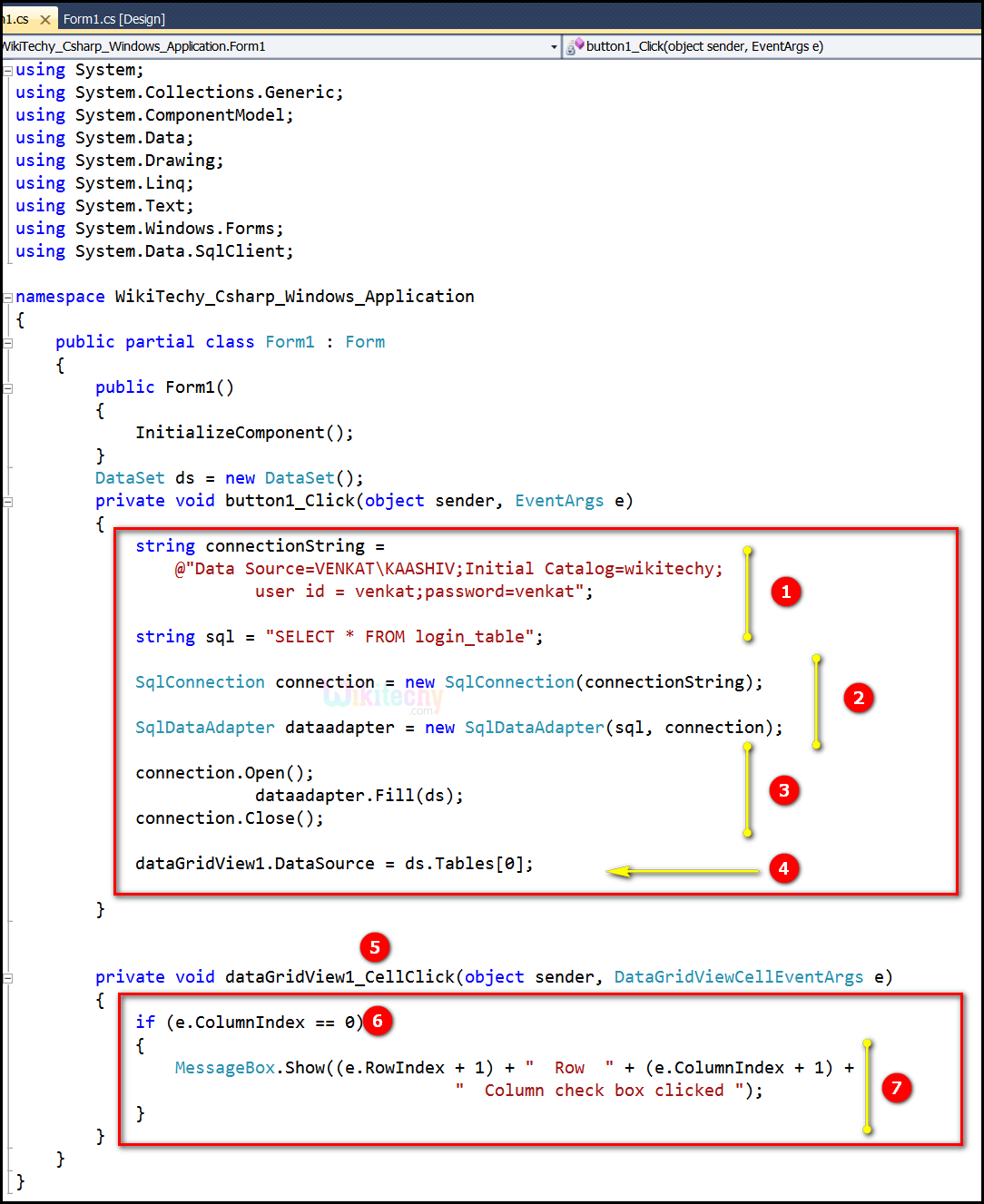
- string connectionString = @"Data Source=VENKAT\KAASHIV;Initial Catalog=wikitechy; user id = venkat;password=venkat"; connection string specifies that includes the source database name here the name is VENKAT\KAASHIV, and other parameters needed to establish the initial catalog connection is wikitechy. The default value is user id and password is venkat. In this example string sql = "SELECT * FROM login_table"; specifies to select the login_table in SQL server.
- SqlConnection connection = new SqlConnection (connectionString); this statement is used to acquire the SqlConnection as a resource. SqlDataAdapter dataadapter = new SqlDataAdapter(sql, connection); specifies to initializes a new instance of the SqlDataAdapter class with a SelectCommand (sql) and a SqlConnection object(connection).
- connection.Open(); specifies to Open the SqlConnection. dataadapter.Fill(ds); specifies to fetches the data from User and fills in the DataSet ds. connection.Close(); it is used to close the Database Connection.
- dataGridView1.DataSource = ds.Tables[0]; is used to specify the data source of datagridview and the table which will be bind to. When we run the code a window will show with a datagridview that containing all the returning rows.
- private void dataGridView1_CellClick(object sender, DataGridViewCellEventArgs e) click event handler for Cellclick specifies to select a row by click a cell on the datagridview. The two parameters, "object sender" and "EventArgs e" parameters of function of event of a button such as private void button2_Click(object sender, EventArgs e).
- if (e.ColumnIndex == 0) specifies to gets the column index of the current DataGridViewCell.
- MessageBox.Show((e.RowIndex + 1) + " Row " + (e.ColumnIndex + 1) + " Column check box clicked "); displays a message box with the specified string "Column check box Clicked" which presents a message to the user.
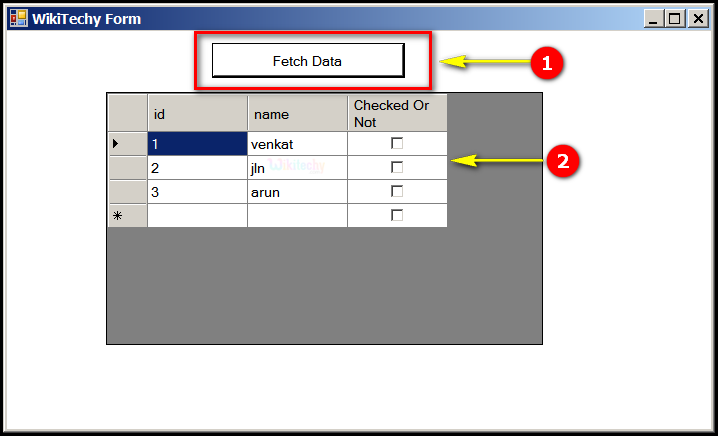
- Here in this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- Here in this output table displays the id "1,2,3", name "venkat, jln, arun" and Checked Or Not "checked box".
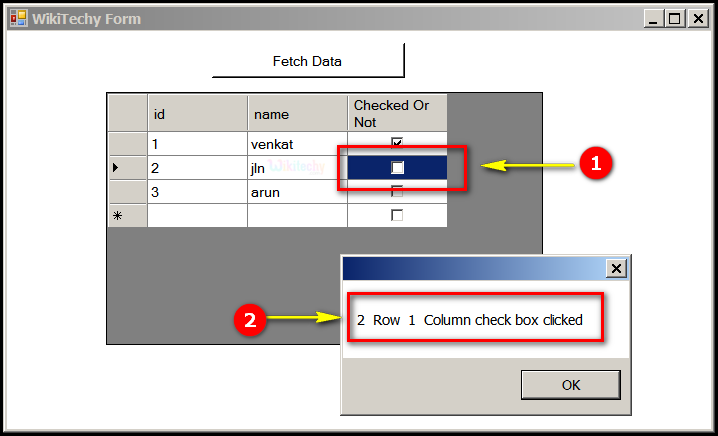
- In this output table displays the id "1,2,3", name "venkat, jln, arun" and Checked or Not "checked box" and select the jln.
- In this output table displays the 2 Row 1 column check box checked message box window is opened.
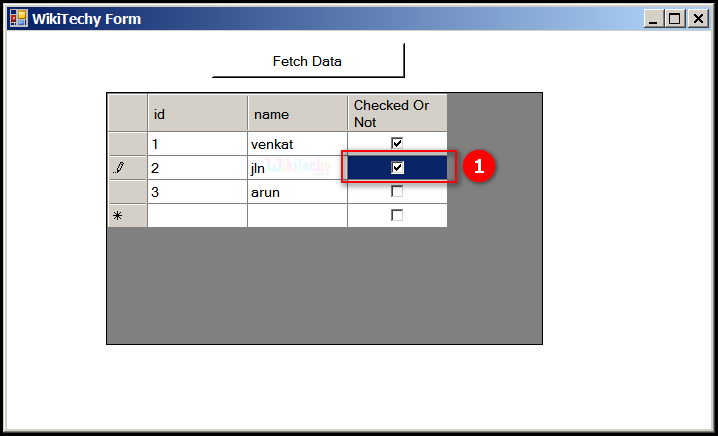
- In this output table displays the id "1,2,3", name "venkat, jln, arun" and Checked or Not "checked box". Here "venkat" and "jln" is checked in table.
Adding check box in datagrid view programmatically
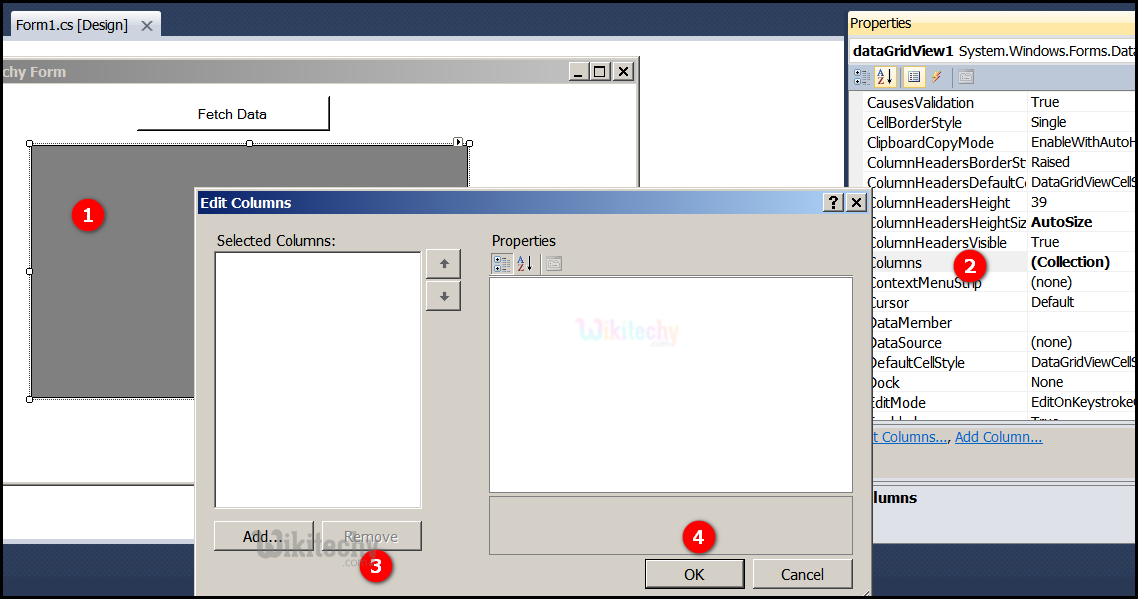
- Go to tool box and click on the DataGridview option the form will be open.
- Go to properties click on column collection pop up Edit Columns window will open after choose the image button.
- Click on "remove" button in Edit Columns window.
- After click on the ok button
Read Also
dot net training institute in chennai tamil nadu , dotnet course in chennai , dot net training institute near meC# Sample Code - C# Examples
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data.SqlClient;
namespace WikiTechy_Csharp_Windows_Application
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
DataSet ds = new DataSet();
private void button1_Click(object sender, EventArgs e)
{
string connectionString =
@"Data Source=VENKAT\KAASHIV;Initial Catalog=wikitechy;
user id = venkat;password=venkat";
string sql = "SELECT * FROM login_table";
SqlConnection connection = new SqlConnection(connectionString);
SqlDataAdapter dataadapter = new SqlDataAdapter(sql, connection);
connection.Open();
dataadapter.Fill(ds);
connection.Close();
dataGridView1.DataSource = ds.Tables[0];
DataGridViewCheckBoxColumn chk = new DataGridViewCheckBoxColumn();
dataGridView1.Columns.Add(chk);
chk.HeaderText = "Check Data";
chk.Name = "chk";
dataGridView1.Rows[2].Cells[2].Value = true;
}
private void dataGridView1_CellClick(object sender, DataGridViewCellEventArgs e)
{
if (e.ColumnIndex == 2)
{
MessageBox.Show((e.RowIndex + 1) + " Row " + (e.ColumnIndex + 1) +
" Column check box clicked ");
}
}
}
}
Code Explanation
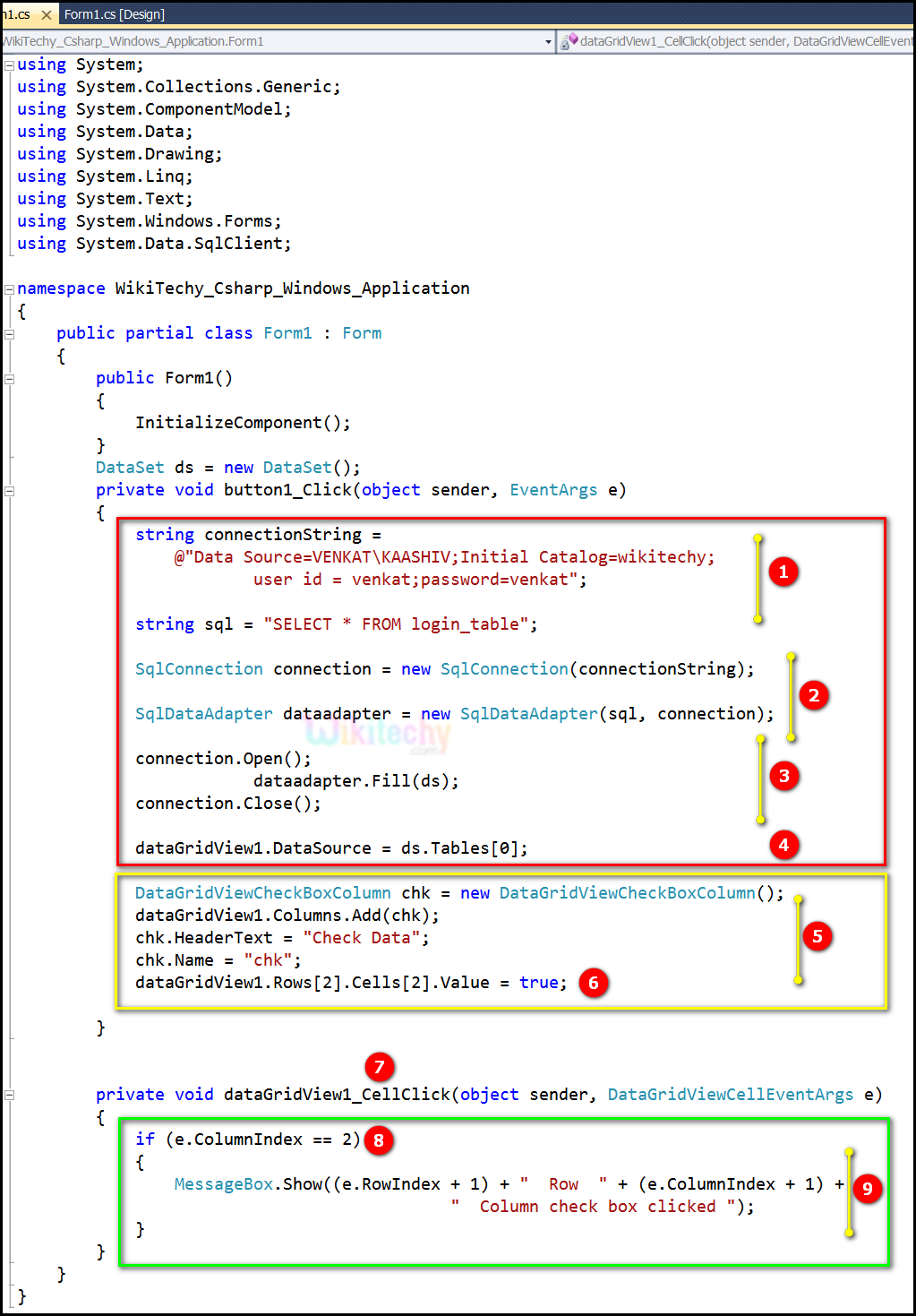
- DataGridViewCheckBoxColumn chk = new DataGridView CheckBoxColumn(); specifies to a new instance of the DataGridViewCheckBoxColumn.
- dataGridView1.Columns.Add(chk); specifies to add check in the column of a DataGridView , the column type contains cells of type DataGridViewCheckColumn.
- chk.HeaderText = "Check Data"; used to store the check data in DataTable.
- Here chk.Name = "chk"; specifies to text series of Unicode name "chk" .
- dataGridView1.Rows[2].Cells[2].Value = true; specifies to modify the value in the second cell of the second row.
- if (e.ColumnIndex == 2) specifies to the column index of the cell that is second formatted.
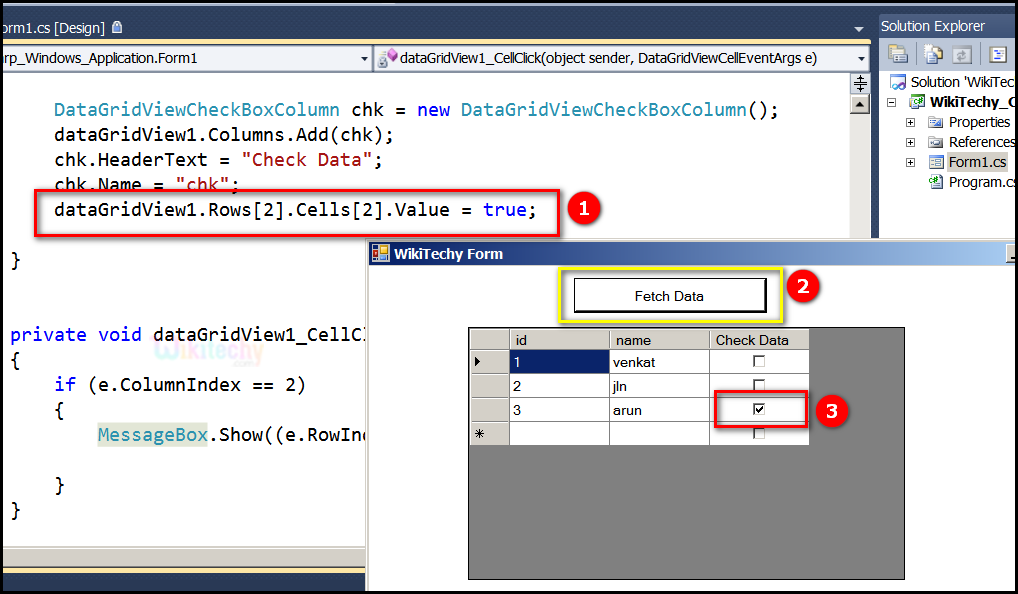
- dataGridView1.Rows[2].Cells[2].Value = true; specifies to modify the value in the second cell of the second row.
- Here in this output the Fetch Data button will display the data values from the SQL and displays it by clicking it.
- In this output table displays the id "1,2,3", name "venkat, jln, arun" and Checked or Not "checked box". Here "arun" data is checked in table.
Sample C# examples - Output :
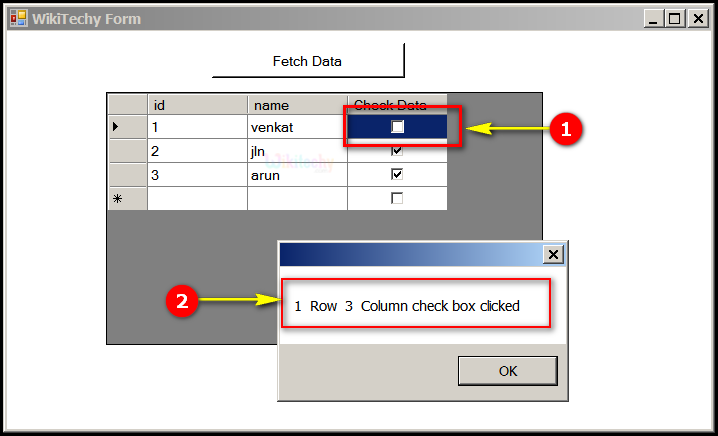
- In this output table displays the id "1,2,3", name "venkat, jln, arun" and Checked or Not "checked box" and select the venkat.
- In this output table displays the 1 Row 3 column check box checked in the message box.